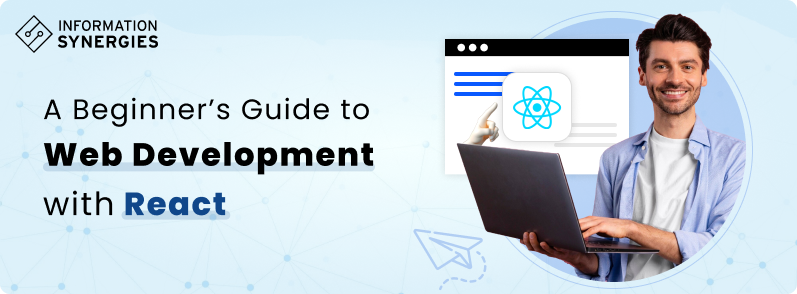
In today’s fast-paced web development landscape, React has emerged as one of the most powerful and popular libraries for building dynamic user interfaces. Whether you’re a beginner or an experienced developer looking to explore React, this guide will provide a solid understanding of what React is, why it was developed, and how to get started with it.
Introduction
React has revolutionized web development, with millions of developers worldwide relying on it to create interactive user interfaces. First released by Facebook in 2013, it has become one of the most popular front-end libraries, trusted by companies like Netflix, Airbnb, and Instagram for their web applications.
It can be overwhelming to dive into the world of React as a beginner. But don’t worry—with the right approach, you can quickly master it and build modern, interactive web applications.
What is React?
React is an open-source JavaScript library primarily used for building user interfaces, particularly single-page applications (SPAs). Its component-based architecture allows developers to create fast, scalable, and dynamic web apps.
React was created to address the challenges of developing dynamic, high-performance applications that must handle frequent changes in the user interface without compromising performance.
Unlike traditional JavaScript frameworks, React focuses solely on the application’s view layer, which means it deals with how the user perceives the application.
Why React Was Developed?
Before React, building interactive UIs for modern web applications involved complex and cumbersome code. Many developers relied on jQuery, but managing and updating the DOM (Document Object Model) became inefficient as apps became more complicated.
Facebook developed React to address this problem. The key motivations for developing React include:
- Improving Performance: React introduced the concept of a Virtual DOM, which allows the library to ender components, improving efficiency and performance.
- React simplifies UI Development by breaking down UI elements into reusable components. This makes it easier for developers to manage large applications.
- Faster Rendering: React’s Virtual DOM ensures that only the changed parts of a UI are updated, reducing unnecessary re-renders and speeding up the application.
- Declarative Syntax: React provides a declarative approach to building UIs, making it easier to understand, debug, and maintain your code.
How React Works?
The core of React’s performance and simplicity is its efficient rendering system, which includes the Virtual DOM and reconciliation process.
- Virtual DOM: React uses a lightweight copy of the actual DOM called the Virtual DOM. When you update your UI, React updates the Virtual DOM first and then compares it to the actual DOM. Only the changed parts are updated, which is much faster than updating the entire DOM.
- Reconciliation is React’s algorithm for comparing the Virtual DOM and the actual DOM. It efficiently determines which parts of the UI need to be updated based on changes in state or props, leading to smoother performance.
- Components: React uses components as the building blocks of an application. Components are reusable and can manage their state and props.
- State and Props: State stores data that changes over time, whereas props pass data from one component to another.
What are Components?
Components are the heart of any React application. They represent a part of the user interface (UI) and are designed to be reusable and modular.
There are two types of components in React:
- Class Components: These are ES6 classes that extend React. Components can have their own state and lifecycle methods. They are less commonly used now but are still essential to React’s history.
- Functional Components: These JavaScript functions accept props as arguments and return a React element. They are more straightforward to write and are now preferred in modern React development, especially with the introduction of Hooks (like useState and useEffect).
Example of a simple, functional component:
jsx
function HelloWorld() {
return <h1>Hello, World!</h1>;
}
What is JSX?
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It is used in React to describe the UI’s appearance.
JSX makes React code more readable and easier to write because it allows you to combine HTML and JavaScript logic in a single file. Even though browsers don’t understand JSX directly, it is compiled into regular JavaScript at build time.
Example of JSX:
const element = <h1>Hello, React!</h1>;
JSX is not required to use React, but it makes the code much cleaner and easier to understand.
One-Way Data Binding
React uses one-way data binding, meaning data flows in a single direction: from parent components to child components via props. This makes React applications more predictable and easier to debug because you know exactly where the data comes from and how it’s being passed around.
One-way data binding makes tracing and managing changes to your app’s state easier. If you need to update data or UI elements, React automatically reflects the changes at the appropriate places in your app.
Getting Started with React
Now that you understand what React is and how it works, it’s time to get started. Here’s a simple guide to setting up React:
- Install Node.js: React requires Node.js and npm (Node Package Manager), so ensure both are installed on your computer.
Create a React App: The easiest way to create a new React project is using the create-react-app command-line tool. Open your terminal and run:
bash
npx create-react-app my-app
cd my-app
npm start
- Explore the Default Project: After running the command above, a fully functional React application will be set up. Explore the src folder to understand the file structure and organization of components.
- Start Building Components: Begin by building small components and gradually increase the complexity of your application.
How a Leading React Development Company Helps
If you’re new to React or don’t have the time to dive deep into it, working with a professional React development company can help you save time and avoid costly mistakes. Here’s how they can assist:
- Custom React Applications: They can build custom, scalable, high-performance React applications tailored to your needs.
- UI/UX Design: Leading React developers will ensure your app works well and provides a great user experience through thoughtful UI/UX design.
- Best Practices: Expert React developers follow best practices, ensuring your app is maintainable, scalable, and efficient.
- Optimization: React development companies can optimize your app for faster performance, reducing load times and ensuring smooth user interactions.
- Ongoing Support: Many React development companies provide continuous support and maintenance, ensuring your app stays up-to-date and bug-free.
Conclusion
React has become one of the most popular and efficient libraries for building modern web applications. By understanding its core concepts, such as components, JSX, one-way data binding, and how it works, you can quickly start building dynamic and interactive applications.
Whether you’re a beginner or looking to enhance your React skills, getting hands-on experience with the library is essential. For more advanced features and optimizations, working with a leading React development company can help you leverage the full potential of React.
Ready to build your first React application or optimize an existing one? Contact us, a leading React development company, for expert advice and top-quality development services tailored to your needs. Let’s bring your web development projects to life with React!